diff --git a/README.md b/README.md
index 46776b9..ff45e67 100644
--- a/README.md
+++ b/README.md
@@ -15,16 +15,16 @@
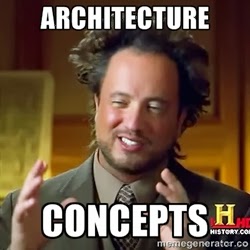
-**WE ARE IN HARDWARE MODE. This project is in a conceptual phase and most stuff does not work yet.**
+**WE ARE IN HARDWARE MODE. This project is in a conceptual phase and most stuff changes weekly. More importantly, it only works against bleeding edge v3 components of Ocean Protocol which are not completely public yet.**
---
**Table of Contents**
- [🏗 Installation](#-installation)
-- [🏄 Usage](#-usage)
- - [1. Providers](#1-providers)
- - [2. Hooks](#2-hooks)
+- [🏄 Quick Start](#-quick-start)
+ - [1. Add Provider](#1-add-provider)
+ - [2. Use Hooks](#2-use-hooks)
- [🦑 Development](#-development)
- [✨ Code Style](#-code-style)
- [👩🔬 Testing](#-testing)
@@ -43,62 +43,25 @@
npm install @oceanprotocol/react
```
-## 🏄 Usage
+## 🏄 Quick Start
-First, wrap your whole app with the [`Web3Provider`](src/providers/Web3Provider) and the [`OceanProvider`](src/providers/OceanProvider).
+### 1. Add Provider
-### 1. Providers
+First, wrap your whole app with the [``](src/providers/OceanProvider).
-```tsx
-import React, { ReactNode } from 'react'
-import { Web3Provider, OceanProvider, Config } from '@oceanprotocol/react'
-
-const config: Config = {
- nodeUri: '',
- aquariusUri: '',
- ...
-}
-
-export default function MyApp({
- children
-}: {
- children: ReactNode
-}): ReactNode {
- return (
-
-
- My App
- {children}
-
- )}
-
- )
-}
-```
-
-The `OceanProvider` requires a Web3 instance to be passed as prop so you can replace the basic [`Web3Provider`](src/providers/Web3Provider) with whatever component/library/provider returning a Web3 instance.
-
-### 2. Hooks
+### 2. Use Hooks
Then within your component use the included hooks to interact with Ocean's functionality. Each hook can be used independently:
```tsx
import React from 'react'
-import {
- useWeb3,
- useOcean,
- useMetadata,
- useConsume
-} from '@oceanprotocol/react'
+import { useOcean, useMetadata, useConsume } from '@oceanprotocol/react'
const did = 'did:op:0x000000000'
export default function MyComponent() {
- // Get web3 from built-in Web3Provider context
- const { web3 } = useWeb3()
-
// Get Ocean instance from built-in OceanProvider context
- const { ocean, account } = useOcean()
+ const { ocean, web3, account } = useOcean()
// Get metadata for this asset
const { title, metadata } = useMetadata(did)
diff --git a/src/providers/OceanProvider/README.md b/src/providers/OceanProvider/README.md
index a268ae5..f983494 100644
--- a/src/providers/OceanProvider/README.md
+++ b/src/providers/OceanProvider/README.md
@@ -2,11 +2,11 @@
The `OceanProvider` maintains a connection to the Ocean Protocol network in multiple steps:
-1. On mount, connect to Aquarius instance right away so any asset metadata can be retrieved before, and independent of any Web3 connections.
-2. Once Web3 becomes available, a connection to all Ocean Protocol network components is established.
-3. Once Ocean becomes available, spits out some info about it.
+1. On mount, setup [Web3Modal](https://github.com/Web3Modal/).
+2. Once connection with Web3Modal is started, Web3 becomes available.
+3. Once Web3 becomes available, connection to Ocean Protocol components are initiated, all available under the `ocean` object.
-Also provides a `useOcean` helper hook to access its context values from any component.
+With the included `useOcean` helper hook you can access all context values from any component.
## Usage
@@ -22,6 +22,12 @@ const config: Config = {
...
}
+const web3ModalOpts = {
+ network: 'mainnet', // optional
+ cacheProvider: true, // optional
+ providerOptions // required
+}
+
export default function MyApp({
children
}: {
@@ -36,6 +42,8 @@ export default function MyApp({
}
```
+The `OceanProvider` uses [Web3Modal](https://github.com/Web3Modal/) to make its initial wallet connection. If you do not pass `web3ModalOpts` as a prop, only the default injected provider will be available. Adding more providers requires you to add them as dependencies to your project and pass them as `providerOptions`. See all the available [Provider Options](https://github.com/Web3Modal/web3modal#provider-options).
+
You can then access the provider context values with the `useOcean` hook:
```tsx